Hi All,
I am using an nrf52832 and using NCS version 2.6.0. I am having an issue where I am trying to initialize and run a thread, but the thread never runs. I have tried an assortment of processes and configurations with no luck thus far. Below will be the relevant code file and an example print output. Any advice on what the issue could be would be greatly appreciated, thanks!
#include <zephyr/sys/util.h> // added from button sample #include <inttypes.h> // added from button sample #include <zephyr/device.h> // added from button sample #include <zephyr/kernel.h> #include <zephyr/drivers/gpio.h> #include <zephyr/sys/printk.h> #include <zephyr/logging/log.h> #include "app_button.h" #include "cab_ble_service.h" #include "main.h" LOG_MODULE_DECLARE(APP_BUTTON); static bool button_press_3s; struct bt_conn *current_connection; #define STACK_SIZE 2048 #define THREAD_PRIORITY 7 K_THREAD_STACK_DEFINE(emergency_noti_thread_stack, STACK_SIZE); struct k_thread emergency_noti_thread_data; struct k_work emergency_noti_work; void send_emergency_noti(void *p1, void *p2, void *p3) { printk("Emergency Noti THREAD FUNCTION EXECUTING\n"); current_connection = Get_My_Connection(); Cab_Send_Emergency_Notification(); } void emergency_noti_work_handler(struct k_work *work) { printk("Work handler invoked, creating and starting thread\n"); k_tid_t tid = k_thread_create(&emergency_noti_thread_data, emergency_noti_thread_stack, STACK_SIZE, send_emergency_noti, NULL, NULL, NULL, THREAD_PRIORITY, 0, K_NO_WAIT); if (tid == NULL) { printk("Failed to create thread\n"); } else { printk("Thread created with id %p, starting thread\n", tid); k_thread_start(tid); printk("Thread start returned\n"); } } static void timer0_cancelled_handler(struct k_timer *dummy) { printk("Timer CANCELLED at %" PRIu32 "\n", k_cycle_get_32()); button_press_3s = false; } static void timer0_expired_handler(struct k_timer *dummy) { button_press_3s = true; printk("Timer EXPIRED at %" PRIu32 "\n", k_cycle_get_32()); Set_Emergency_State(true); int ret = k_work_submit(&emergency_noti_work); if (ret < 0) { printk("Failed to submit work: %d\n", ret); } else { printk("Submitting work\n"); } } static K_TIMER_DEFINE(timer0, timer0_expired_handler, timer0_cancelled_handler); #define BUTTON_0 DT_ALIAS(button0) #if !DT_NODE_HAS_STATUS(BUTTON_0, okay) #error "Unsupported board: button_0 devicetree alias is not defined" #endif static const struct gpio_dt_spec button = GPIO_DT_SPEC_GET_OR(BUTTON_0, gpios, {0}); static struct gpio_callback button_cb_data; void button_event(const struct device *dev, struct gpio_callback *cb, uint32_t pins) { int val = gpio_pin_get_dt(&button); if (val > 0) { printk("Button PRESSED at %" PRIu32 "\n", k_cycle_get_32()); k_timer_start(&timer0, K_MSEC(3000), K_NO_WAIT); printk("Timer START at %" PRIu32 "\n", k_cycle_get_32()); } else { printk("Button RELEASED at %" PRIu32 "\n", k_cycle_get_32()); if (button_press_3s) { // Don't need to stop timer as it should be stopped already // Don't set emergency_state to FALSE } else { k_timer_stop(&timer0); Set_Emergency_State(false); printk("Timer STOP at %" PRIu32 "\n", k_cycle_get_32()); } } } uint8_t Init_App_Button(void) { int ret; if (!gpio_is_ready_dt(&button)) { printk("Error: button device %s is not ready\n", button.port->name); return 0; } ret = gpio_pin_configure_dt(&button, GPIO_INPUT); if (ret != 0) { printk("Error %d: failed to configure %s pin %d\n", ret, button.port->name, button.pin); return 0; } ret = gpio_pin_interrupt_configure_dt(&button, GPIO_INT_EDGE_BOTH); if (ret != 0) { printk("Error %d: failed to configure interrupt on %s pin %d\n", ret, button.port->name, button.pin); return 0; } gpio_init_callback(&button_cb_data, button_event, BIT(button.pin)); gpio_add_callback(button.port, &button_cb_data); printk("Set up button at %s pin %d\n", button.port->name, button.pin); k_work_init(&emergency_noti_work, emergency_noti_work_handler); return 1; }
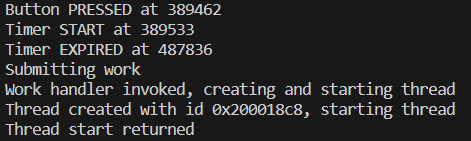
As shown in the above log, thread creation and start appear to be successful, but the thread function send_emergency_noti() never gets called.