Hello,
I'm new to pretty much all of this, but I'll do my best to explain my issue. As the title says, I'm currently trying to get a LSM6DSO sensor to work with the NRF52832 board. I'm using the Nordic sample code for LSM6DSO and using VSCode to build it for the NRF52832. I was having issues with the devicetree not being able to find the sensor using DEVICE_DT_GET_ONE (this function initially returned a negative value), so I added an extra overlay file:
arduino_i2c: &i2c0 {
lsm6dso@6a {
compatible = "st,lsm6dso";
label = "LSM6DSO";
reg = <0x6a>;
};
};
This seemed to fix the issue. The only other thing I changed from the base project code was adding the lines
CONFIG_LOG=y
CONFIG_LOG_PRINTK=y
to my prj.conf file so that I could log any errors.
I've fully connected the sensor to the board and have verified the connections are correct using a colleagues code, so I don't think the connections are the issue. When I build and flash the code, this is the output I get:
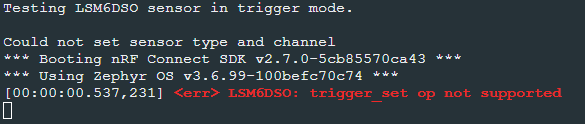
As I said, I'm fairly new to how Nordic organizes code and devicetree, so I'm really just not sure what to do from here. Obviously, the code is running through its main function, but it gets an error when it tries to test the trigger mode. I believe the sample code is supposed to work with an ST board, so I think getting this code to work is just a matter of making small changes to the overlay files or the configuration files, although I could easily be wrong.
Any advice would be greatly appreciated, and let me know if I can provide any more information that might help. Thank you!