In our application build with SDK 2.7.0 on a NRF52840 chip, we get an assert if we update the TX_Power for the connections.
If the bluetooth has made connection, we update the TX power.
}
static void connected(struct bt_conn *conn, uint8_t err) { char addr[BT_ADDR_LE_STR_LEN]; int ret; if (err) { LOG_ERR("Connection failed (err %u)", err); return; } bt_addr_le_to_str(bt_conn_get_dst(conn), addr, sizeof(addr)); LOG_INF("Connected %s", addr); current_conn = bt_conn_ref(conn); LOG_INF("current_conn %d", current_conn); ret = bt_hci_get_conn_handle(current_conn,&conn_handle); LOG_INF("conn_handle %d", conn_handle); if (ret) { LOG_ERR("No connection handle (err %d)\n", ret); } else { set_tx_power(BT_HCI_VS_LL_HANDLE_TYPE_CONN, conn_handle, TX_POWER); } }
static void set_tx_power(uint8_t handle_type, uint16_t handle, int8_t tx_pwr_lvl) { struct bt_hci_cp_vs_write_tx_power_level *cp; struct bt_hci_rp_vs_write_tx_power_level *rp; struct net_buf *buf, *rsp = NULL; int err; buf = bt_hci_cmd_create(BT_HCI_OP_VS_WRITE_TX_POWER_LEVEL, sizeof(*cp)); if (!buf) { LOG_ERR("Unable to allocate command buffer\n"); return; } cp = net_buf_add(buf, sizeof(*cp)); cp->handle = sys_cpu_to_le16(handle); cp->handle_type = handle_type; cp->tx_power_level = tx_pwr_lvl; err = bt_hci_cmd_send_sync(BT_HCI_OP_VS_WRITE_TX_POWER_LEVEL, buf, &rsp); if (err) { uint8_t reason = rsp ? ((struct bt_hci_rp_vs_write_tx_power_level *) rsp->data)->status : 0; LOG_INF("Set Tx power err: %d reason 0x%02x\n", err, reason); return; } rp = (void *)rsp->data; LOG_INF("Actual Tx Power: %d\n", rp->selected_tx_power); net_buf_unref(rsp); }
code works fine if we disable the row: set_tx_power(BT_HCI_VS_LL_HANDLE_TYPE_CONN, conn_handle, TX_POWER);
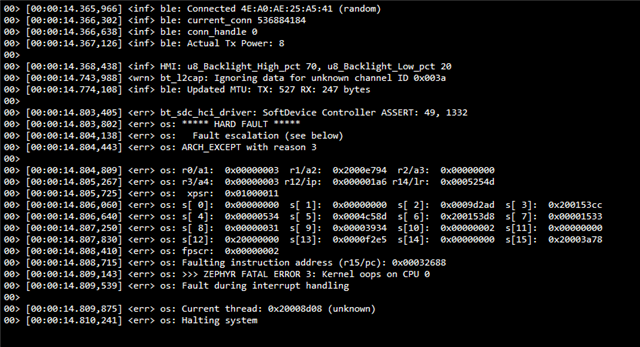