Hello
I am trying to initialize qspi in a ".cpp" file and methods like
PINCTRL_DT_DEFINE(QSPI_NODE); are giving me "out-of-order initializers are nonstandard in C++"
so I had to do this work around
so I had to do this work around
#include <zephyr/drivers/flash.h> #include <zephyr/drivers/pinctrl.h> #include <zephyr/storage/flash_map.h> #include <nrfx_qspi.h> #include <stdint.h> /* Define QSPI node from devicetree */ #define QSPI_NODE DT_NODELABEL(qspi) /* Hardcoded pin configuration */ static const pinctrl_soc_pin_t qspi_pins[] = { NRF_PSEL(QSPI_SCK, 0, 17), // SCK on P0.17 NRF_PSEL(QSPI_IO0, 0, 13), // IO0 on P0.13 NRF_PSEL(QSPI_IO1, 0, 14), // IO1 on P0.14 NRF_PSEL(QSPI_IO2, 0, 15), // IO2 on P0.15 NRF_PSEL(QSPI_IO3, 0, 16), // IO3 on P0.16 NRF_PSEL(QSPI_CSN, 0, 18), // CSN on P0.18 }; /* Define the state for QSPI */ static const struct pinctrl_state qspi_pin_states[] = { { .pins = qspi_pins, .pin_cnt = ARRAY_SIZE(qspi_pins), .id = PINCTRL_STATE_DEFAULT, }, }; /* Define the pinctrl_dev_config for QSPI */ static const struct pinctrl_dev_config qspi_pinctrl_config = { .states = qspi_pin_states, .state_cnt = ARRAY_SIZE(qspi_pin_states), }; /* Configure and initialize QSPI */ static int qspi_init(void) { static const nrfx_qspi_config_t qspi_config = { .prot_if = { .readoc = NRF_QSPI_READOC_READ4IO, .writeoc = NRF_QSPI_WRITEOC_PP4IO, .addrmode = NRF_QSPI_ADDRMODE_24BIT, }, .phy_if = { .sck_delay = 0x05, .dpmen = false, .spi_mode = NRF_QSPI_MODE_0, .sck_freq = NRF_QSPI_FREQ_DIV2, }, .skip_gpio_cfg = true, .skip_psel_cfg = false, }; int ret = pinctrl_apply_state(&qspi_pinctrl_config, PINCTRL_STATE_DEFAULT); if (ret < 0) { LOG_ERR("Failed to apply pinctrl state"); return ret; } nrfx_err_t err = nrfx_qspi_init(&qspi_config, NULL, NULL); if (err != NRFX_SUCCESS) { LOG_ERR("QSPI initialization failed: %d", err); return -EIO; } LOG_INF("QSPI initialized"); return 0; } int qspi_read(uint32_t address, void *data, size_t size) { int ret = nrfx_qspi_read(data, size, address); if (ret != NRFX_SUCCESS) { LOG_ERR("QSPI read failed: %d", ret); return -EIO; } LOG_INF("QSPI read successful"); return 0; } int qspi_write(uint32_t address, const void *data, size_t size) { int ret = nrfx_qspi_write(data, size, address); if (ret != NRFX_SUCCESS) { LOG_ERR("QSPI write failed: %d", ret); return -EIO; } LOG_INF("QSPI write successful"); return 0; }
which builds just fine for me.
however it this method causes the firmware to hault exactly at this line
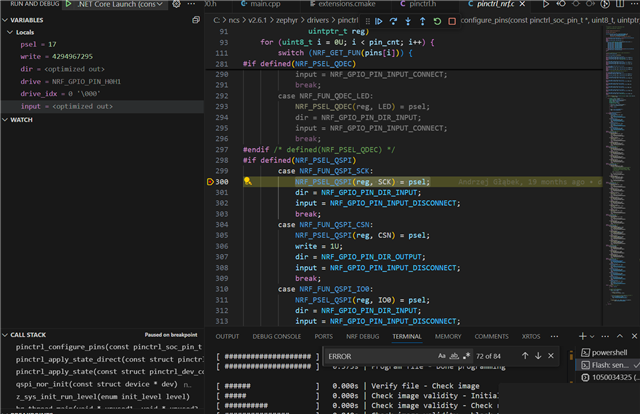
however it this method causes the firmware to hault exactly at this line
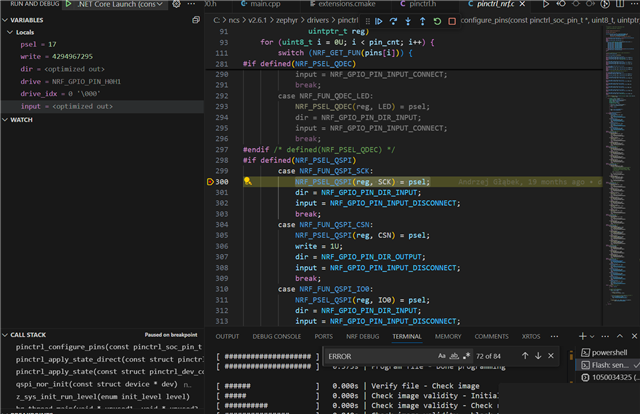
is it a mistake in my initialization ? or does this just not work in cpp?
thank you for your time.
thank you for your time.