I am using VSCode with nRF Connect extension with the 2.9.1 SDK.
I am trying to write my own device driver for the Zephyr OS and the TI CC1101 chip. Since the tutorial isn't up to date yet I am trying to cobble together a lot of different things to make this work.
I have another ticket regarding that setup.
This ticket is about a work around that I found to get past some of those issues and just try to use my driver as a generic SPI device.
I have this in my overlay file:
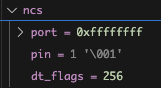