I am trying to interface an SD card with the nRF9160-DK using an SD card adapter module. My goal is to write the string "hello"
to a .txt
file on the SD card. I believe I have correctly connected the SD card module using SPI connections. However, when I run the code, the serial monitor only displays the message "error init"
, indicating that the SD card initialization is
failing. i have provided main code
//main code #include <zephyr/kernel.h> #include <zephyr/device.h> #include <zephyr/drivers/spi.h> #include <zephyr/fs/fs.h> #include <zephyr/storage/disk_access.h> #include <zephyr/logging/log.h> LOG_MODULE_REGISTER(main, LOG_LEVEL_DBG); #define MOUNT_POINT "/SD" #define FILE_PATH MOUNT_POINT "/hello.txt" static struct fs_mount_t fs_mount_sd = { .type = FS_FATFS, .mnt_point = MOUNT_POINT, .fs_data = NULL, .storage_dev = (void *)"SD", }; bool sd_initialized = false; // Function to initialize SPI device const struct device *init_spi_device() { const struct device *spi_dev = DEVICE_DT_GET(DT_NODELABEL(spi2)); if (!spi_dev) { printk("SPI device not found!\n"); } else { printk("SPI device found: %p\n", spi_dev); printk("SPI device name: %s\n", spi_dev->name); } return spi_dev; } // Initialize SD card int initialize_sd_card() { printk("Attempting to initialize SD card...\n"); // Check SPI device availability const struct device *spi_dev = init_spi_device(); if (!spi_dev) { printk("Cannot proceed without SPI device!\n"); sd_initialized = false; return -ENODEV; } int ret = disk_access_init("SD"); if (ret != 0) { printk("SD card initialization failed. Error: %d\n", ret); LOG_WRN("SD card initialization failed. Card may be removed."); sd_initialized = false; return ret; } printk("SD card initialization successful.\n"); ret = fs_mount(&fs_mount_sd); if (ret == 0) { printk("SD card mounted successfully at %s.\n", MOUNT_POINT); LOG_INF("SD card initialized successfully."); sd_initialized = true; return 0; } else { printk("Failed to mount SD card. Error: %d\n", ret); LOG_ERR("Failed to mount SD card: %d", ret); sd_initialized = false; return ret; } } // Write to SD card void write_string_to_file(const char *str) { printk("Preparing to write to file: %s\n", FILE_PATH); struct fs_file_t file; fs_file_t_init(&file); int ret = fs_open(&file, FILE_PATH, FS_O_WRITE | FS_O_CREATE | FS_O_APPEND); if (ret < 0) { printk("Failed to open hello.txt for writing. Error: %d\n", ret); LOG_ERR("Failed to open hello.txt for writing: %d", ret); sd_initialized = false; return; } printk("File opened successfully. Writing data...\n"); ret = fs_write(&file, str, strlen(str)); if (ret < 0) { printk("Failed to write to hello.txt. Error: %d\n", ret); LOG_ERR("Failed to write to hello.txt: %d", ret); } else { printk("Data written successfully to hello.txt: %s\n", str); LOG_INF("Written to hello.txt: %s", str); } fs_close(&file); printk("File closed.\n"); } void main(void) { printk("Starting SD card test with SPI on nRF9160 DK...\n"); if (initialize_sd_card() == 0) { write_string_to_file("Hello, SPI MicroSD!"); } while (1) { if (sd_initialized) { printk("SD card is initialized. Writing data...\n"); write_string_to_file("Hello from Zephyr over SPI!"); } else { printk("SD card not initialized. Retrying...\n"); LOG_WRN("SD card not initialized. Retrying..."); initialize_sd_card(); } k_sleep(K_SECONDS(5)); } }this is prj config file
// prj config CONFIG_SPI=y CONFIG_DISK_ACCESS=y CONFIG_FILE_SYSTEM=y CONFIG_FAT_FILESYSTEM_ELM=y CONFIG_LOG=y CONFIG_DISK_DRIVER_SDMMC=y CONFIG_LOG_DEFAULT_LEVEL=4 CONFIG_SPI_NRFX=y CONFIG_SDHC=ythis is overlay file
//overlay file &spi2 { status = "okay"; compatible = "nordic,nrf-spim"; cs-gpios = <&gpio0 19 GPIO_ACTIVE_LOW>; pinctrl-0 = <&spi1_default>; pinctrl-names = "default"; sdcard: sd@0 { compatible = "zephyr,sdhc-spi-slot"; reg = <0x0>; spi-max-frequency = <8000000>; }; }; &pinctrl { spi1_default:spi1_default{ group0{ psels=<NRF_PSEL(SPIM_MISO,0,16)>, <NRF_PSEL(SPIM_MOSI,0,17)>, <NRF_PSEL(SPIM_SCK,0,18)>; }; }; };this is the error i have been getting
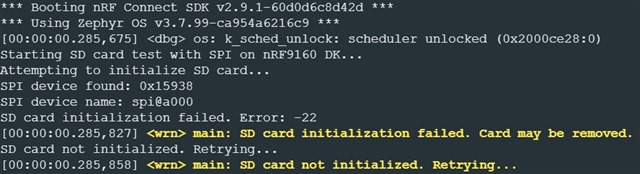