Hello there,
I have used the nRF52840 DK to find the rssi values of all peripheral devices using the interactive example. I would like to create a command to find the rssi value of a single device. I have found the BLE address of the device that I want to use as the peripheral but would like some assistance piecing everything together.
I was thinking of using an if statement to say "if the address is "3E:52:82:FF:88:5F", then print the rssi". I am aware that it is not this straight forward.
My code is as follows and I have included a picture of the command line for the rssi command and the devices command.
Thank you!
static void device_list_print(nrf_cli_t const * p_cli, scanned_device_t * p_device) { for (uint8_t i = 0; i < DEVICE_TO_FIND_MAX; i++) { if (p_device[i].is_not_empty) { nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "Device/RSSI(in dBm): "); char buffer[ADDR_STRING_LEN]; int_addr_to_hex_str(buffer, BLE_GAP_ADDR_LEN, p_device[i].addr); nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "%s %s %d\n", buffer, p_device[i].dev_name, p_device[i].rssi); //address: uint8_t addr[BLE_GAP_ADDR_LEN] //name: dev_name[DEVICE_NAME_MAX_SIZE] } } } static void rssi_print(nrf_cli_t const * p_cli, scanned_device_t * p_device){ for (uint8_t i = 0; i < DEVICE_TO_FIND_MAX; i++) { if (p_device[i].is_not_empty){ if (uint8_t addr[BLE_GAP_ADDR_LEN] == "3E:52:82:FF:88:5F" ) { nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "Device/RSSI(in dBm): "); char buffer[ADDR_STRING_LEN]; int_addr_to_hex_str(buffer, BLE_GAP_ADDR_LEN, p_device[i].addr); nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "%s %s %d\n", buffer, p_device[i].dev_name, p_device[i].rssi); } else { nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "Device/RSSI(in dBm): "); char buffer[ADDR_STRING_LEN]; int_addr_to_hex_str(buffer, BLE_GAP_ADDR_LEN, p_device[i].addr); nrf_cli_fprintf(p_cli, NRF_CLI_NORMAL, "%s %s %d\n", buffer, p_device[i].dev_name, p_device[i].rssi); } } } }
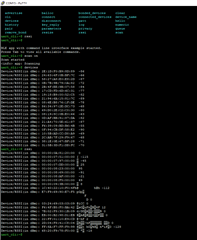