Hi!
I am developing a network of temperature and humidity sensors, using nrf52832 and hts221 with the ide ses ... for this, I am using thingy 52 as a peripheral that sends the data to a central. When trying to read the hts221 sensor, I receive the ERROR 4 on the console. I checked the code again and again, but I can't find out what I'm doing wrong.
I'm using SDK16, thingy 52 1.0.1 and delete the thingy firmware using nrfjrog and then upload my own firmware.
//
// Set up the TWI instance for the HTS221 sensor
//
/* TWI instance ID. */
#define TWI_INSTANCE_ID 0
#define MAX_PENDING_TRANSACTIONS 33
NRF_TWI_MNGR_DEF(m_nrf_twi_mngr, MAX_PENDING_TRANSACTIONS, TWI_INSTANCE_ID);
NRF_TWI_SENSOR_DEF(m_nrf_twi_sensor, &m_nrf_twi_mngr, HTS221_MIN_QUEUE_SIZE);
HTS221_INSTANCE_DEF(m_hts221,&m_nrf_twi_sensor,HTS221_BASE_ADDRESS);
// TWI (with transaction manager) initialization.
static void twi_config(void)
{
uint32_t err_code;
nrf_drv_twi_config_t const config = {
.scl = 8,
.sda = 7,
.frequency = NRF_DRV_TWI_FREQ_100K,
.interrupt_priority = APP_IRQ_PRIORITY_LOWEST,
.clear_bus_init = false
};
NRF_LOG_INFO("TWI HTS221 sensor starting.");
NRF_LOG_FLUSH();
err_code = nrf_twi_mngr_init(&m_nrf_twi_mngr, &config);
APP_ERROR_CHECK(err_code);
err_code = nrf_twi_sensor_init(&m_nrf_twi_sensor);
APP_ERROR_CHECK(err_code);
err_code = hts221_init(&m_hts221);
APP_ERROR_CHECK(err_code);
err_code = hts221_avg_cfg (&m_hts221, HTS221_TEMP_SAMPLES_8, HTS221_HUMIDITY_SAMPLES_16);
APP_ERROR_CHECK(err_code);
}
void m_temp_callback (uint32_t twi_result_t, int16_t * p_rawtemp)
{
NRF_LOG_INFO("RAW TEMP : %d", rawtemp);
temperature = hts221_temp_process(&m_hts221, rawtemp)/8;
}
void m_hum_callback(uint32_t twi_result_h, int16_t * p_rawhum)
{
int tempF;
NRF_LOG_INFO("RAW HUMIDITY : %d\n", rawhum);
humidity = hts221_hum_process(&m_hts221, rawhum)/2;
//
// 2nd task competed
// Temperature and Humidity both processed
// Display results
tempF = temperature*1.8+32;
NRF_LOG_INFO("Temp: %d Degrees C, %d Degrees F", temperature, tempF);
NRF_LOG_INFO("Humidity: %d%%\n", humidity)
}
void read_th()
{
uint32_t err;
// Submit temperature read command to TWI manager
// m_temp_callback called when data is returned
err = hts221_temp_read(&m_hts221, &m_temp_callback , &rawtemp);
APP_ERROR_CHECK(err);
// Submit humidity read command to TWI manager
// m_hum_callback called when data is returned
err = hts221_hum_read(&m_hts221, &m_hum_callback , &rawhum);
APP_ERROR_CHECK(err);
}
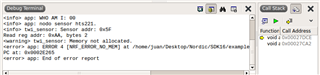