I am currently trying to create a simple BLE observer which listens to advertisement broadcasts from a specific device (given via address). I attached the source code below.
My problem is that I am unable to add the address filter. In more detail: as soon as I run the code on the 5340dk, it logs "<err> logger: Scanning filters cannot be set (err -12)" (see screenshot) which is caused by the failure of the bt_scan_filter_add() function. Does anyone have an idea why this add of the filter may fail? Thanks!
#include <zephyr.h> #include <zephyr/types.h> #include <stddef.h> #include <sys/util.h> #include <sys/byteorder.h> #include <bluetooth/bluetooth.h> #include <bluetooth/uuid.h> #include <bluetooth/gatt.h> #include <bluetooth/hci.h> #include <bluetooth/scan.h> #include <bluetooth/gatt_dm.h> #include <logging/log.h> LOG_MODULE_REGISTER(logger); static void scan_filter_match(struct bt_scan_device_info *device_info, struct bt_scan_filter_match *filter_match, bool connectable) { char addr[BT_ADDR_LE_STR_LEN]; bt_addr_le_to_str(device_info->recv_info->addr, addr, sizeof(addr)); LOG_INF("Filters matched. Address: %s connectable: %d", log_strdup(addr), connectable); } BT_SCAN_CB_INIT(scan_cb, scan_filter_match, NULL, NULL, NULL); static int scan_init(void) { int err; bt_scan_init(NULL); bt_scan_cb_register(&scan_cb); bt_addr_t addr ={ { 0xf0, 0xca, 0xf0, 0xca, 0x00, 0x02 }}; bt_addr_le_t addr_le = { .type = BT_ADDR_LE_PUBLIC, .a = addr }; err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_ADDR, &addr_le); if (err) { LOG_ERR("Scanning filters cannot be set (err %d)", err); return err; } err = bt_scan_filter_enable(BT_SCAN_ADDR_FILTER, false); if (err) { LOG_ERR("Filters cannot be turned on (err %d)", err); return err; } LOG_INF("Scan module initialized"); return err; } void main(void) { /* Initialize the Bluetooth Subsystem */ int err = bt_enable(NULL); if (err) { LOG_ERR("Bluetooth init failed (err %d)\n", err); } LOG_INF("Bluetooth initialized"); /* Start Bluetooth Scanning */ err = scan_init(); if (err) { LOG_ERR("Failed to initialize BLE scan"); } err = bt_scan_start(BT_SCAN_TYPE_SCAN_ACTIVE); if (err) { LOG_ERR("Scanning failed to start (err %d)", err); } LOG_INF("Scanning successfully started"); }
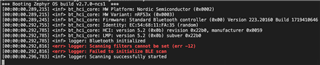