I've been slowly adding NVS functionality to my code. Has been working fine, up until today when I attempted to write a struct I have for storing historical data to flash.
This is the struct:
struct log_data { // data structure to store individual strike events uint8_t strike_level; // SML = 1, MED = 2 or LRG = 4 uint16_t total_strike_count; // Total number of strikes recorded to date struct bt_cts_current_time time_detected; //Time stamp for each strike };
I then have an array of this struct. At this point, MAX_STRIKE_RECORDS = 10, but ultimately I want to see this at 250.
struct log_data lsr_strike_log[MAX_STRIKE_RECORDS];
In my code to read the data in from Flash, I have the following:
uint32_t rc = 0; rc = nvs_read(&fs, HISTORY_ID, &lsr_strike_log, sizeof(lsr_strike_log)); printk("sizeof = %u\n", sizeof(lsr_strike_log)); printk("strike history rc = %u\n", rc);
The return value from nvs_read() is supposed to be the amount of data read in, if everything works as expected. In my case, this should be equal to sizeof(lsr_strike_log), which is 160 bytes. If the return value is larger than this, it apparently means there is more data to read in. This is what I get from my two printk() statements:
sizeof = 160 strike history rc = 4294967294
flash_dev = FLASH_AREA_DEVICE(STORAGE_NODE_LABEL); if (!device_is_ready(flash_dev)) { printk("Flash device %s is not ready\n", flash_dev->name); return -EINVAL; } fs.offset = FLASH_AREA_OFFSET(storage); rc = flash_get_page_info_by_offs(flash_dev, fs.offset, &info); if (rc) { printk("Unable to get page info\n"); return rc; } fs.sector_size = info.size; fs.sector_count = 3U; rc = nvs_init(&fs, flash_dev->name); if (rc) { printk("Flash Init failed\n"); return rc; }
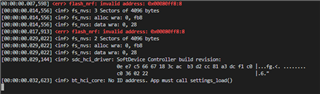