Hi,
I'm trying to test a simple SPI communication between nrf52840 dev board and adxl345. I'm following this guy's youtube tutorial on segger embedded studio. Here's the code to read the device ID and also the hardware setup. I'm getting 242 instead of correct value of 0xe5 or 229. I'm not sure where it's wrong. I tested on an arduino uno and it works. Please help.
Thanks
#include "nrf_drv_spi.h" #include "app_util_platform.h" #include "nrf_gpio.h" #include "nrf_delay.h" #include "boards.h" #include "app_error.h" #include <string.h> #include "nrf_log.h" #include "nrf_log_ctrl.h" #include "nrf_log_default_backends.h" #define ADD_REG_WHO_AM_I 0x00 #define UC_WHO_AM_I_DEFAULT_VALUE 0xE5 #define SPI_BUFSIZE 8 // SPI communication buffer size #define SPI_INSTANCE 0 // SPI instance to be used #define SET_READ_SINGLE_CMD(x) (x | 0x80) // local macro - set read single command (DEPENDS ON SENSOR) uint8_t spi_tx_buf[SPI_BUFSIZE]; // spi tx buffer uint8_t spi_rx_buf[SPI_BUFSIZE]; // spi rx buffer static volatile bool spi_xfer_done; // flag to signal that the SPI instance has completed the transfer static const nrf_drv_spi_t m_spi = NRF_DRV_SPI_INSTANCE(SPI_INSTANCE); // create SPI instance /* SPI Event handler function, on every tranfer this callback function is triggered */ void spi_event_handler(nrf_drv_spi_evt_t const *p_event, void *p_context){ spi_xfer_done = true; } /* Initialize the spi instance */ void spi_init(void){ // Create a sturct to hold the configurations and set these values to default */ nrf_drv_spi_config_t spi_config = NRF_DRV_SPI_DEFAULT_CONFIG; // Assign pins to SPI instance spi_config.ss_pin = 47; spi_config.miso_pin = 46; spi_config.mosi_pin = 45; spi_config.sck_pin = 44; // Configure the transfer speed by setting the clock for data transmission spi_config.frequency = NRF_DRV_SPI_FREQ_4M; /* Call the SPI initialization function within APP_ERROR_CHECK function so that if any error occurs during initialization then we can get the response on debug window */ APP_ERROR_CHECK(nrf_drv_spi_init(&m_spi, &spi_config, spi_event_handler, NULL)); } int read_reg(int reg){ spi_tx_buf[0] = SET_READ_SINGLE_CMD(reg); // set the read command for reading a single byte spi_xfer_done = false; // reset the flag APP_ERROR_CHECK(nrf_drv_spi_transfer(&m_spi, spi_tx_buf, 2, spi_rx_buf, 2)); // call the spi transfer function while(spi_xfer_done == false){}; // wait till the transfer is done return spi_rx_buf[1]; // return the data } int main(void) { int intRegValue; APP_ERROR_CHECK(NRF_LOG_INIT(NULL)); // initialize the logger module and check if any error occurred during initialization NRF_LOG_DEFAULT_BACKENDS_INIT(); // initialize the default backends for nrf logger spi_init(); // call the SPI initialization function NRF_LOG_INFO("APP STARTED"); intRegValue = read_reg(ADD_REG_WHO_AM_I); // get the returned value NRF_LOG_INFO("this came up = %d", intRegValue); if(intRegValue == UC_WHO_AM_I_DEFAULT_VALUE){ NRF_LOG_INFO("Correct data!"); }else{ NRF_LOG_INFO("INCORRECT"); } while (1) { } }
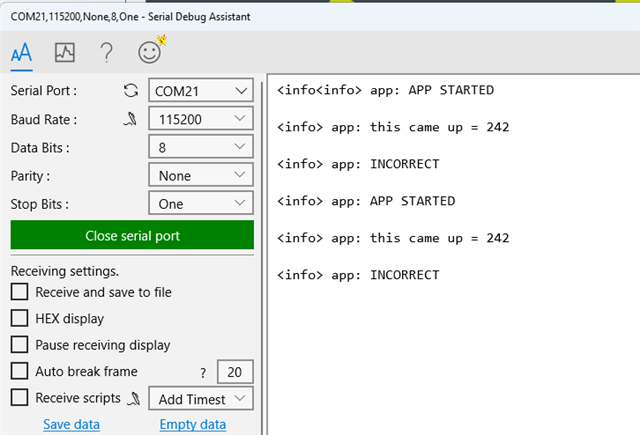