From my understanding I am limited to the software PWM API from nRF Connect SDK (using v2.3.0) - nordic,nrf-sw-pwm
printf("DTS sw-pwm driver name: %s\n", (DEVICE_DT_GET(DT_NODELABEL(sw_pwm)))->name); uint64_t cycles; pwm_get_cycles_per_sec((DEVICE_DT_GET(DT_NODELABEL(sw_pwm))), 0, &cycles); printf("pwm cycles per sec: %lld\n", cycles);
pwm cycles per sec: 16000000 // not validated this value but seems consistent & code compiles so...
Is there any documentation or another project I can reference as I can only find code that uses pwm-leds. Ideally I am looking to bypass modules like pwm-leds and control the sw-pwm channels from application code
nrf52820.dtsi
sw_pwm: sw-pwm { compatible = "nordic,nrf-sw-pwm"; status = "disabled"; generator = <&timer2>; clock-prescaler = <0>; #pwm-cells = <3>; };
&sw_pwm { status ="okay"; channel-gpios = <&gpio0 15 GPIO_ACTIVE_HIGH>, <&gpio0 16 GPIO_ACTIVE_LOW>; clock-prescaler = <0>; };
CONFIG_PWM=y CONFIG_DT_HAS_NORDIC_NRF_SW_PWM_ENABLED=y
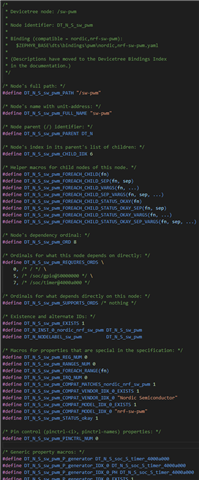