Edit: evidently "struct k_thread my_thread_data_1;" has to be defined outside of the function? That seems to solve the problem, although "struct k_thread my_thread_data_0;" works inside main()... If that is correct, then I solved the problem but I am surprised "struct k_thread" can be defined both inside and outside of a function, sometimes work and sometimes not?
I am able to create and start a thread from main(), but when I start a identical thread from a function I get an error?
It seems happy to create the thread in the function as long as I don't start it.
I chose to use k_thread_create() instead of K_THREAD_DEFINE() because
- I don't need the thread unless an input matches a select-case statement.
- Eventually, I wish to reuse the thread after is has expired...
But it there is a better way of doing this I am open to suggestions.
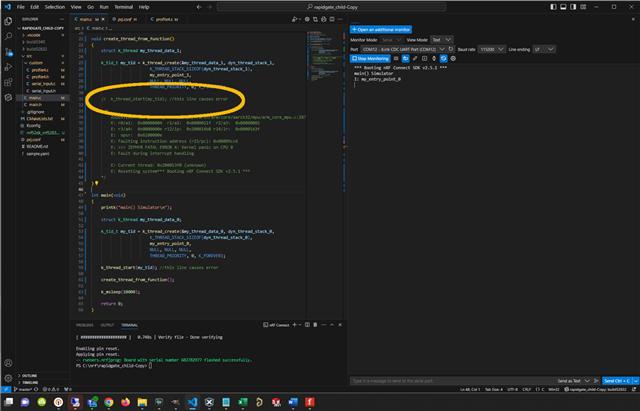
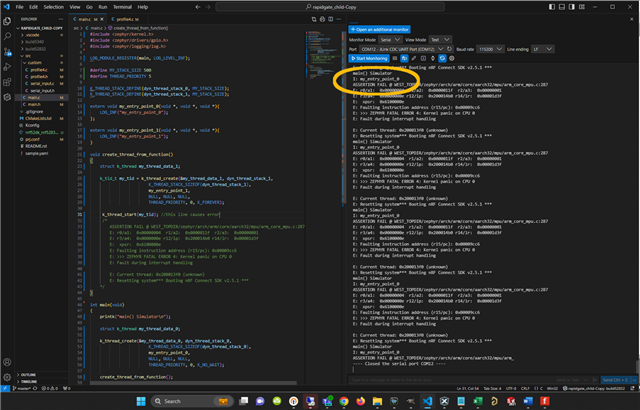
#include <zephyr/kernel.h> #include <zephyr/drivers/gpio.h> #include <zephyr/logging/log.h> LOG_MODULE_REGISTER(main, LOG_LEVEL_INF); #define MY_STACK_SIZE 500 #define THREAD_PRIORITY 5 K_THREAD_STACK_DEFINE(dyn_thread_stack_0, MY_STACK_SIZE); K_THREAD_STACK_DEFINE(dyn_thread_stack_1, MY_STACK_SIZE); extern void my_entry_point_0(void *, void *, void *){ LOG_INF("my_entry_point_0"); }; extern void my_entry_point_1(void *, void *, void *){ LOG_INF("my_entry_point_1"); } void create_thread_from_function() { struct k_thread my_thread_data_1; k_tid_t my_tid = k_thread_create(&my_thread_data_1, dyn_thread_stack_1, K_THREAD_STACK_SIZEOF(dyn_thread_stack_1), my_entry_point_1, NULL, NULL, NULL, THREAD_PRIORITY, 0, K_FOREVER); k_thread_start(my_tid); //this line causes error /* ASSERTION FAIL @ WEST_TOPDIR/zephyr/arch/arm/core/aarch32/mpu/arm_core_mpu.c:287 E: r0/a1: 0x00000004 r1/a2: 0x0000011f r2/a3: 0x00000001 E: r3/a4: 0x0000000e r12/ip: 0x200014b0 r14/lr: 0x00001d3f E: xpsr: 0x6100000e E: Faulting instruction address (r15/pc): 0x00009cc6 E: >>> ZEPHYR FATAL ERROR 4: Kernel panic on CPU 0 E: Fault during interrupt handling E: Current thread: 0x200013f0 (unknown) E: Resetting system*** Booting nRF Connect SDK v2.5.1 *** */ } int main(void) { printk("main() Simulator\n"); struct k_thread my_thread_data_0; k_thread_create(&my_thread_data_0, dyn_thread_stack_0, K_THREAD_STACK_SIZEOF(dyn_thread_stack_0), my_entry_point_0, NULL, NULL, NULL, THREAD_PRIORITY, 0, K_NO_WAIT); create_thread_from_function(); k_msleep(10000); return 0; }