Hi
I tried to put descriptor on the characteristic recently and it didn't seem to work. Is there something wrong with that?
/*Initialize alert service parameter and add charactoristics to the service*/ uint32_t ble_alert_init(ble_nus_t * p_alert, ble_nus_init_t const * p_alert_init) { uint32_t err_code; ble_uuid_t ble_uuid; ble_uuid128_t alert_base_uuid = ALERT_BASE_UUID; VERIFY_PARAM_NOT_NULL(p_alert); VERIFY_PARAM_NOT_NULL(p_alert_init); /*Initialize the second custom service structure.*/ p_alert->data_handler = p_alert_init->data_handler; err_code = sd_ble_uuid_vs_add(&alert_base_uuid, &p_alert->uuid_type); //VERIFY_SUCCESS(err_code); ble_uuid.type = p_alert->uuid_type; ble_uuid.uuid = BLE_UUID_ALERT_SERVICE; /*Add second custom service.*/ err_code = sd_ble_gatts_service_add(BLE_GATTS_SRVC_TYPE_PRIMARY, &ble_uuid, &p_alert->service_handle); VERIFY_SUCCESS(err_code); /**@snippet [Adding proprietary characteristic to the SoftDevice] */ ble_add_char_params_t add_char_params; ble_add_char_user_desc_t user_desc_config_struct; ble_add_char_user_desc_t user_desc_alert_struct; // Initialize and add the Configuration Characteristic memset(&add_char_params, 0, sizeof(add_char_params)); add_char_params.uuid = BLE_UUID_NUS_CONFIG_CHARACTERISTIC; add_char_params.uuid_type = p_alert->uuid_type; add_char_params.max_len = sizeof(uint8_t); add_char_params.init_len = sizeof(uint8_t); add_char_params.is_var_len = false; add_char_params.p_init_value = &Threshold; add_char_params.char_props.read = 1; add_char_params.char_props.write_wo_resp = 1; add_char_params.read_access = SEC_OPEN; add_char_params.write_access = SEC_OPEN; static char user_desc_config[] = "CONFIGURATION"; user_desc_config_struct.p_char_user_desc = (uint8_t*)user_desc_config; user_desc_config_struct.max_size = strlen(user_desc_config); user_desc_config_struct.size = strlen(user_desc_config); user_desc_config_struct.is_var_len = false; add_char_params.p_user_descr = &user_desc_config_struct; err_code = characteristic_add(p_alert->service_handle, &add_char_params, &p_alert->tx_handles); if (err_code != NRF_SUCCESS) { return err_code; } // Initialize and add the Alert Characteristic uint8_t sampledata[] = "HELLO_LUMOS"; memset(&add_char_params, 0, sizeof(add_char_params)); add_char_params.uuid = BLE_UUID_NUS_ALERT_CHARACTERISTIC; add_char_params.uuid_type = p_alert->uuid_type; add_char_params.max_len = sizeof(sampledata); add_char_params.init_len = strlen((char*)sampledata); add_char_params.is_var_len = true; add_char_params.p_init_value = sampledata; add_char_params.char_props.notify = 1; add_char_params.char_props.write_wo_resp = 1; add_char_params.read_access = SEC_OPEN; add_char_params.write_access = SEC_OPEN; add_char_params.cccd_write_access = SEC_OPEN; static char user_desc_alert[] = "ALERTS"; user_desc_alert_struct.p_char_user_desc = (uint8_t*)user_desc_alert; user_desc_alert_struct.max_size = strlen(user_desc_alert); user_desc_alert_struct.size = strlen(user_desc_alert); add_char_params.p_user_descr = &user_desc_alert_struct; err_code = characteristic_add(p_alert->service_handle, &add_char_params, &p_alert->rx_handles); return err_code; }
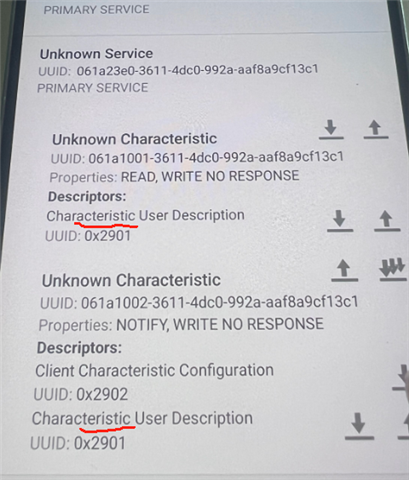
If I take the following code that adds characteristic, descriptor will be displayed successfully,I want to know why my code above won't display descriptor.
/*Initialize alert service parameter and add charactoristics to the service*/ uint32_t ble_alert_init(ble_nus_t * p_alert, ble_nus_init_t const * p_alert_init) { uint32_t err_code; ble_uuid_t ble_uuid; uint8_t value[130] = {0x00}; uint8_t data_len = 0; ble_uuid128_t alert_base_uuid = ALERT_BASE_UUID; VERIFY_PARAM_NOT_NULL(p_alert); VERIFY_PARAM_NOT_NULL(p_alert_init); /*Initialize the second custom service structure.*/ p_alert->data_handler = p_alert_init->data_handler; err_code = sd_ble_uuid_vs_add(&alert_base_uuid, &p_alert->uuid_type); //VERIFY_SUCCESS(err_code); ble_uuid.type = p_alert->uuid_type; ble_uuid.uuid = BLE_UUID_ALERT_SERVICE; /*Add second custom service.*/ err_code = sd_ble_gatts_service_add(BLE_GATTS_SRVC_TYPE_PRIMARY, &ble_uuid, &p_alert->service_handle); VERIFY_SUCCESS(err_code); /**@snippet [Adding proprietary characteristic to the SoftDevice] */ ble_gatts_char_md_t char_md; ble_gatts_attr_md_t cccd_md; ble_gatts_attr_t attr_char_value; ble_gatts_attr_md_t attr_md; memset(&cccd_md, 0, sizeof(cccd_md)); BLE_GAP_CONN_SEC_MODE_SET_OPEN(&cccd_md.read_perm); BLE_GAP_CONN_SEC_MODE_SET_OPEN(&cccd_md.write_perm); cccd_md.vloc = BLE_GATTS_VLOC_STACK; static char *text1 = "CONFIGUARTION"; memset(&char_md, 0, sizeof(char_md)); char_md.char_props.read = 1; char_md.char_props.write_wo_resp = 1; char_md.p_char_user_desc = (uint8_t*) text1; char_md.char_user_desc_size = strlen(text1); char_md.char_user_desc_max_size = strlen(text1); char_md.p_char_pf = NULL; char_md.p_user_desc_md = NULL; char_md.p_cccd_md = &cccd_md; char_md.p_sccd_md = NULL; memset(&attr_md, 0, sizeof(attr_md)); BLE_GAP_CONN_SEC_MODE_SET_OPEN(&attr_md.read_perm); BLE_GAP_CONN_SEC_MODE_SET_OPEN(&attr_md.write_perm); attr_md.vloc = BLE_GATTS_VLOC_STACK; attr_md.rd_auth = 0; attr_md.wr_auth = 0; attr_md.vlen = 1; memset(&attr_char_value, 0, sizeof(attr_char_value)); /* Add config char */ ble_uuid.type = p_alert->uuid_type; ble_uuid.uuid = BLE_UUID_NUS_CONFIG_CHARACTERISTIC; data_len = 1; /*Get Device configuration Length.*/ attr_char_value.p_uuid = &ble_uuid; attr_char_value.p_attr_md = &attr_md; attr_char_value.init_len = data_len; attr_char_value.init_offs = 0; attr_char_value.max_len = data_len; attr_char_value.p_value = &Threshold; //printf("\nThreshold %d\n",Threshold); sd_ble_gatts_characteristic_add(p_alert->service_handle, &char_md, &attr_char_value, &p_alert->tx_handles); // Initialize and add the Configuration Characteristic static char *text2 = "ALERTS"; memset(&char_md, 0, sizeof(char_md)); char_md.char_props.notify = 1; char_md.char_props.write_wo_resp = 1; char_md.p_char_user_desc = (uint8_t *)text2; //NULL; char_md.char_user_desc_size = strlen(text2); char_md.char_user_desc_max_size = strlen(text2); ble_uuid.uuid = BLE_UUID_NUS_ALERT_CHARACTERISTIC; memset(&cccd_md, 0, sizeof(cccd_md)); cccd_md.vloc = BLE_GATTS_VLOC_STACK; BLE_GAP_CONN_SEC_MODE_SET_OPEN(&cccd_md.read_perm); BLE_GAP_CONN_SEC_MODE_SET_OPEN(&cccd_md.write_perm); attr_md.vloc = BLE_GATTS_VLOC_STACK; attr_md.rd_auth = 1; attr_md.wr_auth = 0; attr_md.vlen = 1; memset(&attr_char_value, 0, sizeof(attr_char_value)); memset(value, 0x00, sizeof(value)); char *sampledata = "HELLO_LUMOS"; data_len = strlen(sampledata); attr_char_value.p_uuid = &ble_uuid; attr_char_value.p_attr_md = &attr_md; attr_char_value.init_len = data_len; attr_char_value.init_offs = 0; attr_char_value.max_len = strlen(sampledata); attr_char_value.p_value = (uint8_t*)sampledata; return sd_ble_gatts_characteristic_add(p_alert->service_handle, &char_md, &attr_char_value, &p_alert->rx_handles); }
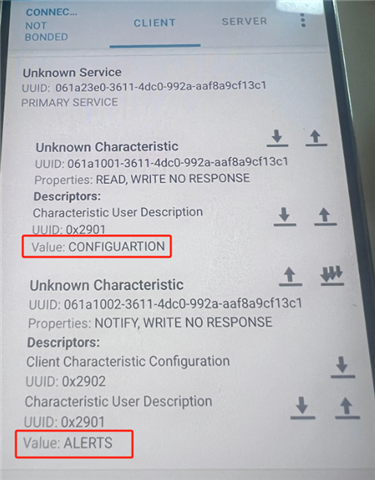
Best regard