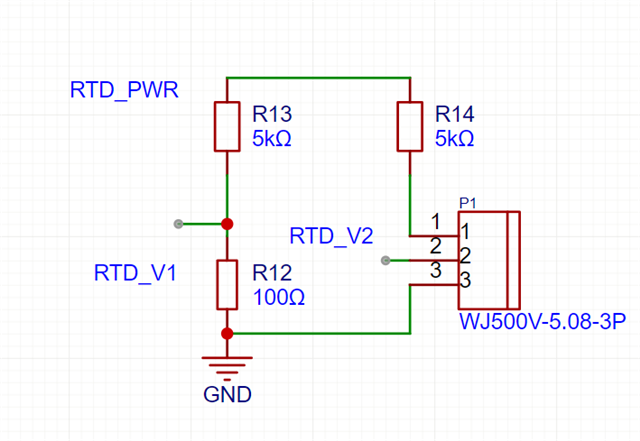
channel@4 { reg = <4>; zephyr,gain = "ADC_GAIN_1_6"; zephyr,reference = "ADC_REF_INTERNAL"; zephyr,acquisition-time = <ADC_ACQ_TIME_DEFAULT>; zephyr,input-positive = <NRF_SAADC_AIN4>; /* P0.03 */ zephyr,input-negative = <NRF_SAADC_AIN6>; /* P0.03 */ zephyr,resolution = <12>; };
#if !DT_NODE_EXISTS(DT_PATH(zephyr_user)) || \ !DT_NODE_HAS_PROP(DT_PATH(zephyr_user), io_channels) #error "No suitable devicetree overlay specified" #endif #define DT_SPEC_AND_COMMA(node_id, prop, idx) \ ADC_DT_SPEC_GET_BY_IDX(node_id, idx), static const struct adc_dt_spec adc_chan4 = ADC_DT_SPEC_GET_BY_IDX(DT_PATH(zephyr_user), 1); static const struct gpio_dt_spec rtd = GPIO_DT_SPEC_GET(DT_PATH(zephyr_user), rtd_gpios); int main(void) { int err; uint32_t count = 0; uint16_t buf_1, buf_2; struct adc_sequence sequence_1 = { .buffer = &buf_1, /* buffer size in bytes, not number of samples */ .buffer_size = sizeof(buf_1),}; int rc; rc = gpio_pin_configure_dt(&rtd, GPIO_OUTPUT); if (rc < 0) { printf("Could not configure sw0 GPIO (%d)\n", rc); return 0; } gpio_pin_set_dt(&rtd, 0); if (!adc_is_ready_dt(&adc_chan4)) { printk("ADC controller device %s not ready\n", adc_chan4.dev->name); return 0; } err = adc_channel_setup_dt(&adc_chan4); if (err < 0) { printk("Could not setup channel (%d)\n", err); return 0; } ; while (1) { int32_t val_mv_1 = 0; (void)adc_sequence_init_dt(&adc_chan4, &sequence_1); err = adc_read_dt(&adc_chan4, &sequence_1); if (err < 0) { printk("Could not read (%d)\n", err); continue; } val_mv_1 = (int32_t)((int16_t)buf_1); err = adc_raw_to_millivolts_dt(&adc_chan4, &val_mv_1); if (err < 0) { printk(" (value in mV not available)\n"); } else { printk(" = %d mV\n", val_mv_1); } k_msleep(1000); } return 0; }
00> = -9 mV
00> = 10 mV
00> = -13 mV
00> = -9 mV
00> = -4 mV
00> = -8 mV
00> = 0 mV
00> = -6 mV
00> = -4 mV
00> = -2 mV
00> = 1 mV