How do I fix it until I send the STOP bit completely?
{
uint32_t timeout;
uint32_t start_tick;
uint32_t current_tick;
uint8_t sleep = 0;
if(len == 0)
return;
timeout = TIMEOUT_TICKS*len;
start_tick = cnt_get();
// check sleep or not
if(mUartProcessing == 0)
{
uart_wakeup();
sleep = 1;
}
NRF_GPIO->OUTSET = ((uint32_t)1 << PIN_UART_SENDING);
NRF_UART0->TASKS_STARTTX = 1;
for(uint16_t i=0; i<len; i++)
{
NRF_UART0->TXD = data[i];
while(NRF_UART0->EVENTS_TXDRDY==0)
{
//
current_tick = cnt_get();
if( cnt_diff_compute(current_tick, start_tick) > timeout)
{
NRF_UART0->EVENTS_TXDRDY = 0;
NRF_UART0->TASKS_STOPTX = 1;
NRF_GPIO->OUTCLR = ((uint32_t)1 << PIN_UART_SENDING);
return;
}
}
NRF_UART0->EVENTS_TXDRDY = 0;
}
NRF_UART0->TASKS_STOPTX = 1;
NRF_GPIO->OUTCLR = ((uint32_t)1 << PIN_UART_SENDING);
if(sleep)
uart_sleep();
}
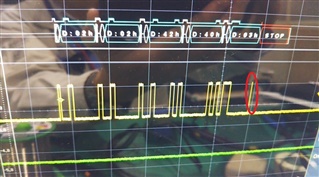