Hi,
I'm trying to make a script in Python using the nRF52840 dongle, to make some BLE advertising.
I'm using:
- Python 3.7.2 (32 bit)
- pc_ble_driver_py library
- Windows 10
- Visual Code
- nRF52840 dongle with USB CDC ACM FW
Now I would like to set a name to my device as I can see it through nRF APP on my mobile phone.
Searching through similar issues in other posts, I've found this function sd_ble_gap_device_name_set, which defined as follow:
def sd_ble_gap_device_name_set(adapter, p_write_perm, p_dev_name, len): return _nrf_ble_driver_sd_api_v2.sd_ble_gap_device_name_set(adapter, p_write_perm, p_dev_name, len)
To figure out how to use it, I've searched in some tutorials and found Bluetooth low energy Advertising, a beginner's tutorial which are written in C;
The function in C is declared as follow:
uint32_t sd_ble_gap_device_name_set ( ble_gap_conn_sec_mode_t const * p_write_perm, uint8_t const * p_dev_name, uint16_t len )
In my script, the function is used as follow:
# Device Name Change device_name = split_str(DEVICE_NAME) gap_device_name = [hex(ord(x)) for x in device_name] str_length = len(DEVICE_NAME.encode(encoding='utf_8')) driver.sd_ble_gap_device_name_set( peripheral.adapter.driver.rpc_adapter, None, DEVICE_NAME.encode(encoding='utf_8'), str_length )
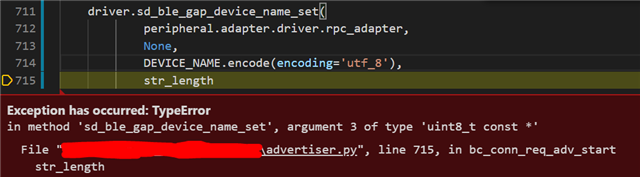