Hi,
Zephyr 3.0 , nrf5340 ncs 1.9.1
i can successfully sent data with
int adxl362ext_get_fifo_data(const struct device *dev,uint8_t *read_buf, uint16_t count,struct k_poll_signal *signal_) { const struct adxl362ext_config *cfg = dev->config; int err; uint8_t access[1] = { ADXL362EXT_READ_FIFO}; const struct spi_buf buf[2] = { { .buf = access, .len = 1 }, { .buf = read_buf, .len = count } }; struct spi_buf_set tx = { .buffers = buf, .count = 1 }; const struct spi_buf_set rx = { .buffers = buf, .count = 2 }; err = spi_transceive_dt(&cfg->bus,&tx, &rx); //err = spi_transceive_async_dt(&cfg->bus,&tx, &rx,signal_); return err; }
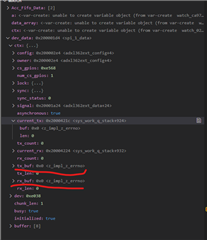
static inline int spi_transceive_async_dt(const struct spi_dt_spec *spec, const struct spi_buf_set *tx_bufs, const struct spi_buf_set *rx_bufs,struct k_poll_signal *signal_) { return spi_transceive_async(spec->bus, &spec->config, tx_bufs, rx_bufs,signal_); }
void spi_context_update_rx(struct spi_context *ctx, uint8_t dfs, uint32_t len) { #ifdef CONFIG_SPI_SLAVE if (spi_context_is_slave(ctx)) { ctx->recv_frames += len; } #endif /* CONFIG_SPI_SLAVE */ if (!ctx->rx_len) { return; } if(len>100){ volatile int oo; oo=0; } if (len > ctx->rx_len) { LOG_ERR("Update exceeds current buffer"); return; } ctx->rx_len -= len; if (!ctx->rx_len) { /* Current buffer is done. Get the next one to be processed. */ ++ctx->current_rx; --ctx->rx_count; ctx->rx_buf = (uint8_t *) spi_context_get_next_buf(&ctx->current_rx, &ctx->rx_count, &ctx->rx_len, dfs); } else if (ctx->rx_buf) { ctx->rx_buf += dfs * len; } LOG_DBG("rx buf/len %p/%zu", ctx->rx_buf, ctx->rx_len); }
if(len>100){ volatile int oo; oo=0; }
uint8_t access[1] = { ADXL362EXT_READ_FIFO}; const struct spi_buf buf[2] = { { .buf = access, .len = 1 }, { .buf = read_buf, .len = 256 } }; struct spi_buf_set tx = { .buffers = buf, .count = 1 }; const struct spi_buf_set rx = { .buffers = buf, .count = 2 }; #if(CONFIG_SPI_ASYNC) err = spi_transceive_async_dt(&cfg->bus,&tx, &rx,signal_); #else