Hi
We are using 52833 adc, but the actual value of the adc is not within our expected value. We want to know the adc accuracy, can you give us some advice? Thanks
sdk: sdk17.1.0
adc: 12bit NRF_SAADC_REFERENCE_INTERNAL NRF_SAADC_GAIN1_4
But it seem no effect,the adc value changes of ain0 and ain1 are not synchronized sometimes.
R = 27kΩ * (ain1adc / ain0adc -ain1adc)
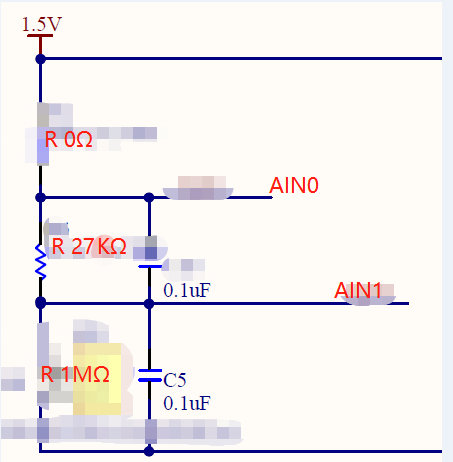