Hi everyone
I am testing the modbus library over zephyr framework (Nrfconnect (skd v2.2.0 ) on visual studio code).
I started from the examples (link) and I am implementing a modbus master.
In the image below you can see, by status analyzer, the TX and DE pin before conversion to RS-485.
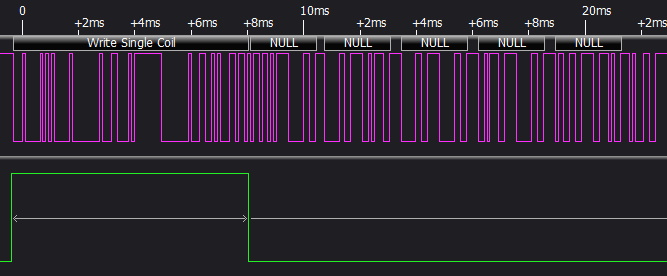
#include <zephyr/kernel.h> #include <zephyr/sys/util.h> #include <zephyr/drivers/gpio.h> #include <zephyr/modbus/modbus.h> #include <zephyr/logging/log.h> LOG_MODULE_REGISTER(mbc_sample, LOG_LEVEL_INF); static int client_iface; const static struct modbus_iface_param client_param = { .mode = MODBUS_MODE_RTU, .rx_timeout = 50000, .serial = { .baud = 9600, .parity = UART_CFG_PARITY_NONE, .stop_bits_client = UART_CFG_STOP_BITS_1, }, }; #define MODBUS_NODE DT_COMPAT_GET_ANY_STATUS_OKAY(zephyr_modbus_serial) static int init_modbus_client(void) { const char iface_name[] = {DEVICE_DT_NAME(MODBUS_NODE)}; client_iface = modbus_iface_get_by_name(iface_name); int lres = 0; return modbus_init_client(client_iface, client_param); } void main(void) { static uint8_t client = 1; int coil = 12; int err; bool stato = false; init_modbus_client(); bool todo = true; //node,coil,val modbus_write_coil(client_iface, 4, 12, true); }
So I am trying to set coil 12 of slave 4 to true.
In modbus term this mean:
FC=5
ID=4
VALUE=FF00
CRC=6C4C
so needed symbol that have to be send di uart are: 40-5000-12-FF00-6C4C.
Via logic analyzer i verified that the trasmission is correct except that some bit are sent (number and shape change every time).
In a nutshell, I have verified that the modbus stack works perfectly, i.e., from the set coil command I get the correct modbus sequence and the same data is copied with a memcopy into the buffer of the uarte. the problem is that the uarte transmits other symbols that I do not understand by whom they are being queued.
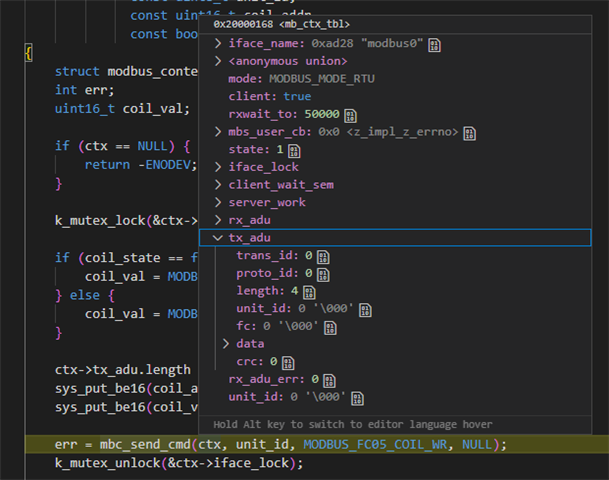
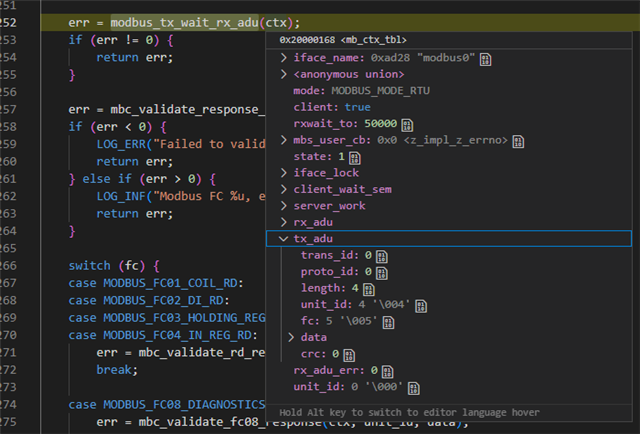
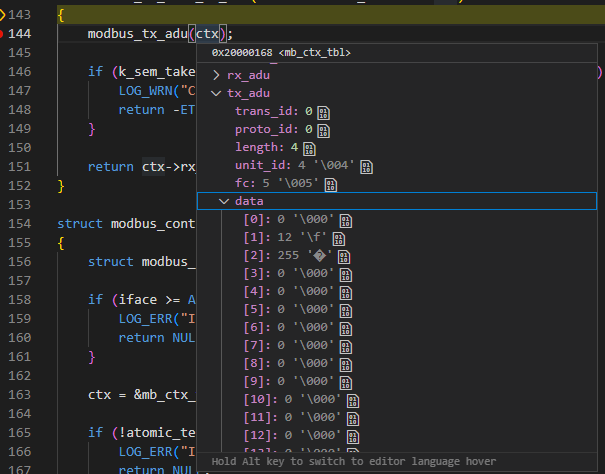
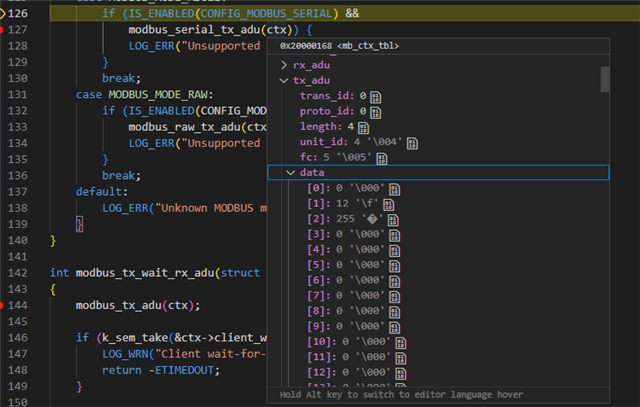
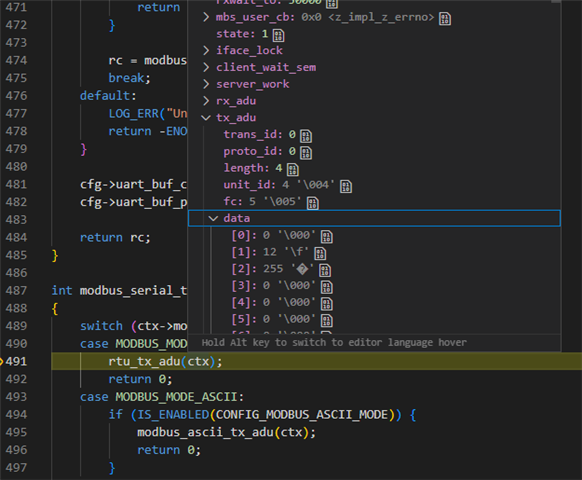
cfg->uart_buf[0] = ctx->tx_adu.unit_id; cfg->uart_buf[1] = ctx->tx_adu.fc; tx_bytes = 2 + ctx->tx_adu.length; data_ptr = &cfg->uart_buf[2]; memcpy(data_ptr, ctx->tx_adu.data, ctx->tx_adu.length); ctx->tx_adu.crc = crc16_ansi(&cfg->uart_buf[0], ctx->tx_adu.length + 2); sys_put_le16(ctx->tx_adu.crc, &cfg->uart_buf[ctx->tx_adu.length + 2]); tx_bytes += 2; cfg->uart_buf_ctr = tx_bytes; cfg->uart_buf_ptr = &cfg->uart_buf[0]; LOG_HEXDUMP_DBG(cfg->uart_buf, cfg->uart_buf_ctr, "uart_buf"); LOG_DBG("Start frame transmission"); modbus_serial_rx_off(ctx); modbus_serial_tx_on(ctx);
modbus_serial_rx_off(ctx); modbus_serial_tx_on(ctx);
uart_irq_tx_enable(cfg->dev);
z_impl_uart_irq_tx_enable(dev);
api->irq_tx_enable(dev);
Maybe it's just a matter of setting something up correctly.
Can you help me with this?
In my opinion it is something at the uarte level