hi,
i am trying to connect peripherials ( blood pressure, pulseox meter) with nrf5340 using uuid and mac, initially using uuid filter are used to scan the devices around and store the mac of the device. once the mac is stored we use address filter to filter the device based on the mac address that was stored earlier.
once the mac address matches with the stored mac address the connect with the device is established and gatt discovery is initialed and the handles are collected and data is received and subscribing to them. everything works as expected. and we could get the vitals from the blood pressure and pulse oximeter.
but we face an unexpected controller reset all of sudden in between in a random pattern whenever the scan_start function is initialed. since we use more than one device we initial Scan_start to initiate the filers to scan for other devices this is done during the connected, disconnected and once gatt_ discovery call back function is succeeded. the nrf controller gets reset automatically during the scan_start function at any on the given call back function.
static void set_scan_filters(void) { // scan filters are determined based on the current mode (pairing or connecting) // and based on which (if any) connections are currently active int err; // remove existing filters //printk("Resetting scan filters\n"); bt_scan_filter_remove_all(); if(hub_mode == HUB_MODE_PAIRING_MODE) { // in Pairing mode, we want to just filter by UUID printk("Setting new scan filters for pairing mode based on UUIDs and current active connections\n"); if(p_blood_pres_conn == NULL) { // not currently connected to any BP sensor, so add that filter //printk("Setting scan filter for BP UUID\n"); err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_UUID, BT_UUID_BPS); } if(p_pulse_ox_conn == NULL) { // not currently connected to any Pulse Ox sensor, so add that filter //printk("Setting scan filter for Pulse Ox UUID\n"); err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_UUID, BT_UUID_PUL_SERVICE); } if(p_therm_conn == NULL) { // not currently connected to any Thermometer, so add that filter //printk("Setting scan filter for Thermometer UUID\n"); err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_UUID, BT_UUID_TEMP_SERVICE); } err = bt_scan_filter_enable(BT_SCAN_UUID_FILTER, false); if (err) { printk("Filters cannot be turned on (err %d)\n", err); } } else if(hub_mode == HUB_MODE_CONNECT_MODE) { // in Connect mode, we want to connect only if we have been given a target address and are // not currently connected to that type of device printk("Setting new scan filters for connecting mode based on target addresses and current active connections\n"); if(p_blood_pres_conn == NULL) { // not currently connected to any BP sensor // check if we have a specific address to connect to if(have_target_blood_pres_address == true) { // Have a target address - set filter //printk("Setting scan filter for target BP address\n"); bt_addr_le_t addr_le_bps; memset(&addr_le_bps, 0x00, sizeof(addr_le_bps)); memcpy(addr_le_bps.a.val, target_blood_pres_address, sizeof(target_blood_pres_address)); addr_le_bps.type = BT_ADDR_LE_PUBLIC; err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_ADDR, &addr_le_bps); } printk( "Not reseted yet in Bp condition"); } if(p_pulse_ox_conn == NULL) { // not currently connected to any pulse ox // check if we have a specific address to connect to if(have_target_pulse_ox_address == true) { // Have a target address - set filter //printk("Setting scan filter for target Pulse Ox address\n"); bt_addr_le_t addr_le_pulseox; memset(&addr_le_pulseox, 0x00, sizeof(addr_le_pulseox)); memcpy(addr_le_pulseox.a.val, target_pulse_ox_address, sizeof(target_pulse_ox_address)); addr_le_pulseox.type = BT_ADDR_LE_PUBLIC; err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_ADDR, &addr_le_pulseox); } printk( "Not reseted yet in plus condition"); } if(p_therm_conn == NULL) { // not currently connected to any thermometer // check if we have a specific address to connect to if(have_target_therm_address == true) { // Have a target address - set filter //printk("Setting scan filter for target Thermometer address\n"); bt_addr_le_t addr_le_therm; memset(&addr_le_therm, 0x00, sizeof(addr_le_therm)); memcpy(addr_le_therm.a.val, target_therm_address, sizeof(target_therm_address)); addr_le_therm.type = BT_ADDR_LE_PUBLIC; err = bt_scan_filter_add(BT_SCAN_FILTER_TYPE_ADDR, &addr_le_therm); } printk( "Not reseted yet in threm condition"); } err = bt_scan_filter_enable(BT_SCAN_ADDR_FILTER, false); if (err) { printk("Filters cannot be turned on (err %d)\n", err); } printk( "Not reseted yet in connetcion function"); } } static int scan_start(void) { int err; uint8_t chan_map[5] = { 0x55, 0x55, 0, 0, 0}; err = bt_le_set_chan_map(chan_map); if (err) { printk("Bad Channel (err %d)\n", err); } // always refresh scan filters before starting scanning set_scan_filters(); printk("Starting scanning\n"); err = bt_scan_start(BT_SCAN_TYPE_SCAN_ACTIVE); if (err) { // error printk("Scanning failed to start (err %d)\n", err); //Scan Error uint16_t scan_error_payload = SCAN_ERROR_PAYLOAD; send_message_data(SCAN_ERROR, &scan_error_payload, sizeof(scan_error_payload)); } else { // success hub_scan_init_state = HUB_SCANNING; } return err; }
we suspected it might be due to the stack overflow and we tried to increase the size however stil the issue sustained. we are not sure what the issue is since we could not find any debug error in the debugger kindly help us how to identify the issue related to the rest.
# disable DCDC CONFIG_BOARD_ENABLE_DCDC_APP=n CONFIG_BOARD_ENABLE_DCDC_NET=n CONFIG_BOARD_ENABLE_DCDC_HV=n # Enable the UART driver # CONFIG_UART_ASYNC_API=y CONFIG_UART_INTERRUPT_DRIVEN=y CONFIG_NRFX_UARTE0=y CONFIG_SERIAL=y CONFIG_CONSOLE=y CONFIG_UART_CONSOLE=y # Enable the BLE stack with GATT Client configuration CONFIG_BT=y CONFIG_BT_CENTRAL=y CONFIG_BT_SMP=y CONFIG_BT_GATT_CLIENT=y CONFIG_BT_MAX_CONN=20 CONFIG_BT_MAX_PAIRED=20 CONFIG_BT_CONN_CTX=y CONFIG_BT_SMP_APP_PAIRING_ACCEPT=y # Enable the BLE modules from NCS CONFIG_BT_NUS_CLIENT=n CONFIG_BT_SCAN=y CONFIG_BT_SCAN_FILTER_ENABLE=y CONFIG_BT_SCAN_UUID_CNT=3 CONFIG_BT_SCAN_ADDRESS_CNT=3 CONFIG_BT_GATT_DM=y CONFIG_BT_GATT_DM_MAX_ATTRS=60 CONFIG_HEAP_MEM_POOL_SIZE=8192 # This example requires more stack CONFIG_SYSTEM_WORKQUEUE_STACK_SIZE=16384 CONFIG_MAIN_STACK_SIZE= 4096 # Enable bonding CONFIG_BT_SETTINGS=y CONFIG_FLASH=y CONFIG_FLASH_PAGE_LAYOUT=y CONFIG_FLASH_MAP=y CONFIG_NVS=y CONFIG_SETTINGS=y # Config logger CONFIG_RTT_CONSOLE=y CONFIG_LOG=y CONFIG_USE_SEGGER_RTT=y CONFIG_LOG_BACKEND_RTT=y CONFIG_LOG_BACKEND_UART=n CONFIG_LOG_PRINTK=n CONFIG_ASSERT=y CONFIG_BT_DEBUG_LOG=y CONFIG_DK_LIBRARY=y CONFIG_NO_OPTIMIZATIONS=y #Debug Keys. MAKE SURE TO SET THESE TO n FOR PRODUCTION CONFIG_BT_USE_DEBUG_KEYS=y CONFIG_BT_STORE_DEBUG_KEYS=y CONFIG_WATCHDOG=n
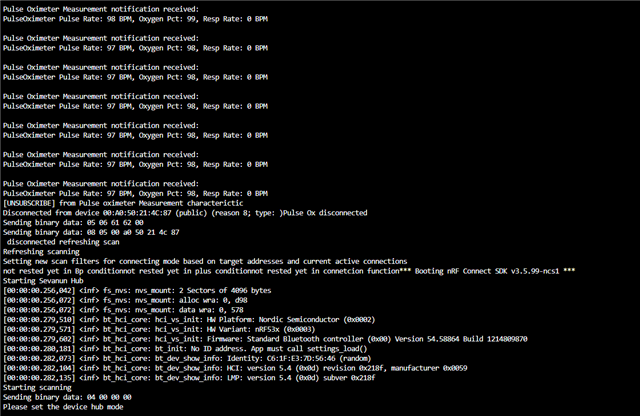